Developers across the globe are always on the lookout for quick and efficient ways to build applications with robust APIs, and Python developers are no exception. With the rise in web application development and the need for scalable backend services, having a solid understanding of building REST APIs is crucial.
In this guide, we will walk through the process of creating a dynamic REST API with Flask—Python’s minimalist web framework that packs a powerful punch.
Building a REST API with Flask is not only rewarding but also educational. You’ll learn about different HTTP methods and how to handle them using Flask, all while following the RESTful design principles. By the end, you’ll be equipped with the knowledge to develop APIs tailored to your application’s needs.
Why Build REST APIs with Flask?
Flask is a minimalist yet powerful Python web framework that provides the flexibility developers need to build scalable APIs. Its simplicity, combined with an active plugin ecosystem, makes it perfect for rapid development. With Flask, you can:
- Handle HTTP Protocols: Implement functionalities like GET, POST, PUT, and DELETE with minimal setup.
- Follow REST Principles: Create clean, logical, and future-proof APIs.
- Leverage a Lightweight Framework: Focus on your application rather than boilerplate code.
Preparing Your Environment
Before we begin crafting the API, there are a few prerequisites we need to have in place:
Basic understanding of Python: Being comfortable with Python syntax and concepts is necessary.
Understanding HTTP Protocol and REST: It is essential to have a foundational understanding of the HTTP methods(GET, POST, PUT, DELETE) and REST architecture.
Familiarity with Postman: We will test our API endpoints using Postman, an API development environment. If you haven’t used it before, now is a great time to get acquainted with it.
Flask and Pip Installed: Ensure you have Flask installed. If not, you can run pip install flask
.
Once your environment is set up, you’re ready to start crafting your Flask REST API. Python virtual environment is also recommended for better project management and dependency control.
Creating the Base Project
The first step in our API creation process is to set up the base project with Flask. We’ll create a simple Hello, World! application to test our API is working as expected. Here’s how you can do it:
First, start by creating a project directory and navigating inside it. Then, create a new Python file named app.py
and add the following code:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
By running python app.py
from your terminal, you should see that your Flask application is up and running.
HTTP Methods for API
REST APIs heavily rely on the principles of REST – Representational State Transfer. A key concept in REST is the use of HTTP methods to specify the intent of the request. These HTTP methods are:
GET: Used to indicate a read operation. It should not change the server’s state.
POST: Used to create a new resource(s) or submit data to the server.
PUT: Used to update an existing resource, or if it does not exist, then create one.
DELETE: Used to delete the specified resource.
We will now modify our app.py
to handle these methods.
Example of HTTP Method Using Flask
Here’s a quick example of using HTTP methods in a Flask REST API. Suppose we want to create a route for a ‘books’ resource.
We use the @app.route
decorator with the default “GET” argument to handle GET requests that list all books. For instance,
@app.route('/books', methods=['GET'])
def get_books():
# Implementation to fetch and return all books
pass
When handling POST requests that add a new book, we use the @app.route
decorator with the “POST” argument, like so:
@app.route('/books', methods=['POST'])
def add_book():
# Implementation to append a new book to the list
pass
The same applies to PUT and DELETE requests, where you supply “PUT” and “DELETE” as the arguments.
Writing the Code for a Book API
We will write the code to handle each HTTP method for our Book API. Below is an example of implementing a RESTful API for managing books.
from flask import Flask,jsonify
app = Flask(__name__)
books = []
# GET - to get all books
@app.route('/books', methods=['GET'])
def get_books():
return jsonify({'books': books})
# POST - to add a new book
@app.route('/books', methods=['POST'])
def add_book():
new_book = request.get_json()
books.append(new_book)
return jsonify({'message': 'Book added successfully!'})
# PUT - to update a book
@app.route('/books/<string:title>', methods=['PUT'])
def update_book(title):
for book in books:
if book['title'] == title:
book['author'] = request.get_json().get('author')
book['read'] = request.get_json().get('read')
return jsonify({'message': 'Book updated successfully!'})
return jsonify({'message': 'Book not found!'})
# DELETE - to remove a book
@app.route('/books/<string:title>', methods=['DELETE'])
def delete_book(title):
for book in books:
if book['title'] == title:
books.remove(book)
return jsonify({'message': 'Book deleted successfully!'})
return jsonify({'message': 'Book not found!'})
if __name__ == '__main__':
app.run(debug=True)
In the above code, we maintain a list called books
, which acts as our database
. Each book in the list is a dictionary with keys like “title”, “author”, and “read”.
Learn: Why Python virtual environments are important?
Running the Code
Save your app.py
and run it with python app.py
. Your server should now be listening on localhost
at port 5000
(https://localhost:5000/). Now, you can move on to the next step—running and testing the API with Postman.
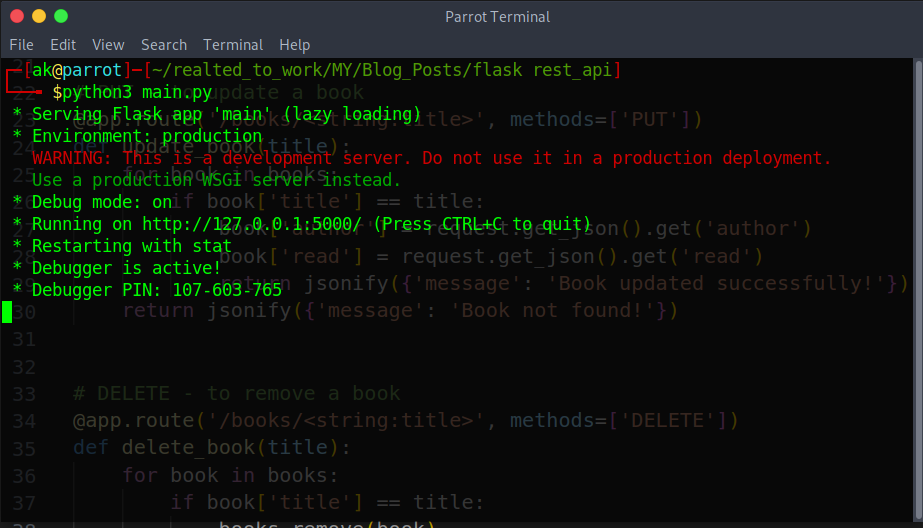
Testing the API with Postman
Postman is a popular tool for API development and an essential part of the workflow.
First, open Postman and create a new request using the appropriate method, URL, and any necessary request body or parameters.
Send the request and observe the response.
For ‘GET’ requests, you’ll receive a list of books.
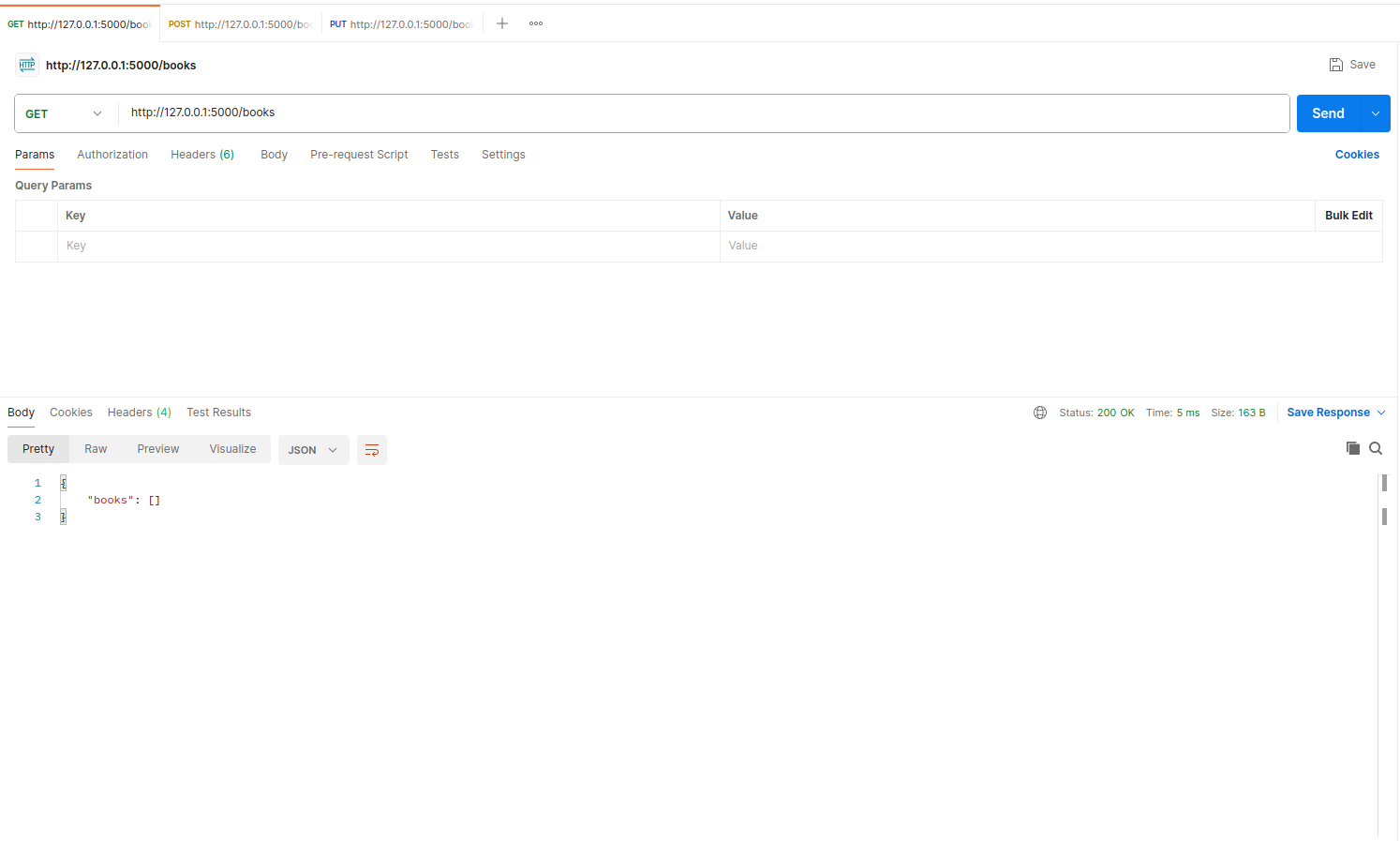
For ‘POST’ requests, add a new book by sending a JSON object in the request body, similar to.
{
"title": "New Book",
"author": "Your Name",
"read": true
}
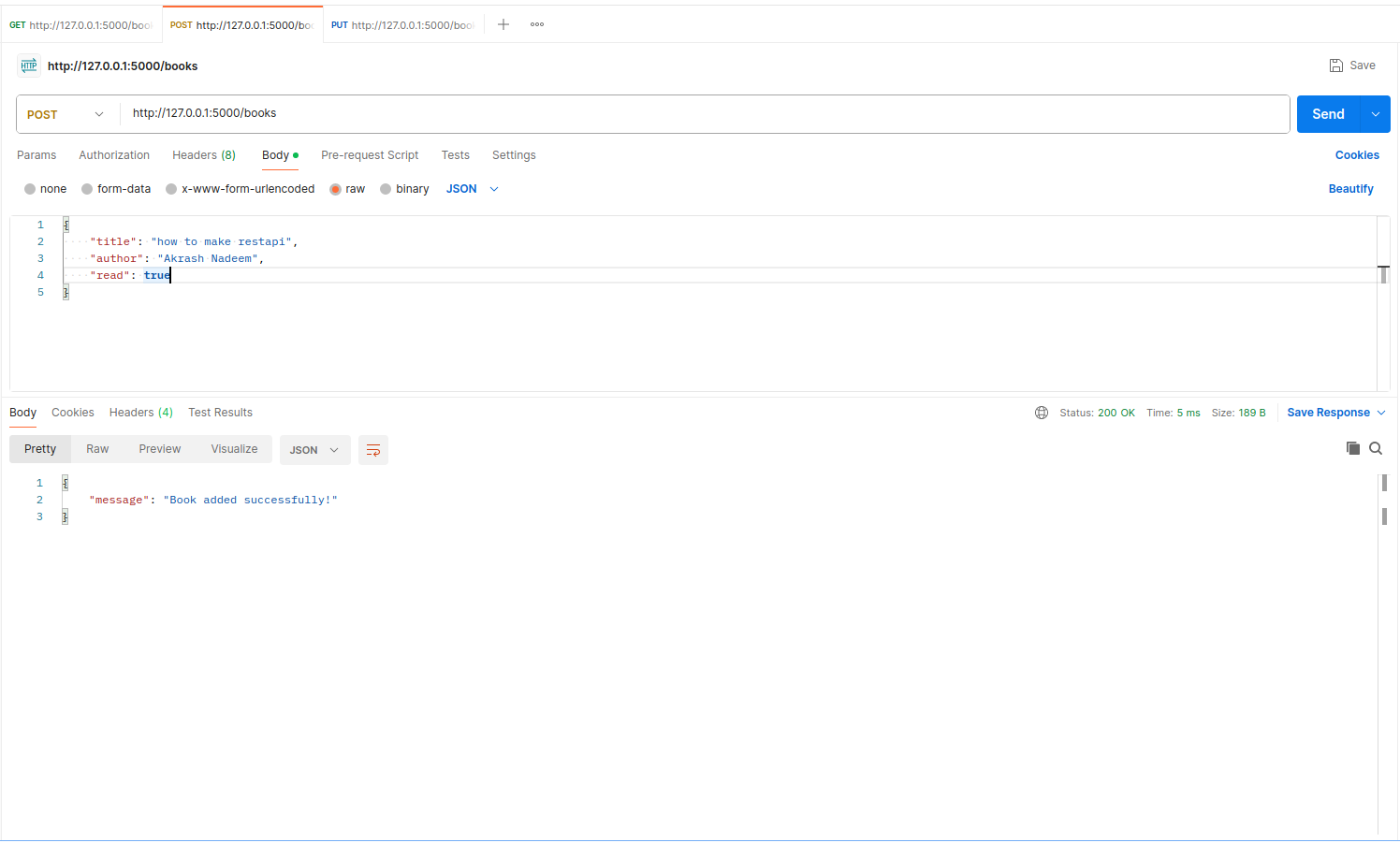
For ‘PUT’ requests, update an existing book by specifying the ‘title’ in the endpoint URL and providing the updated book information in the request body.
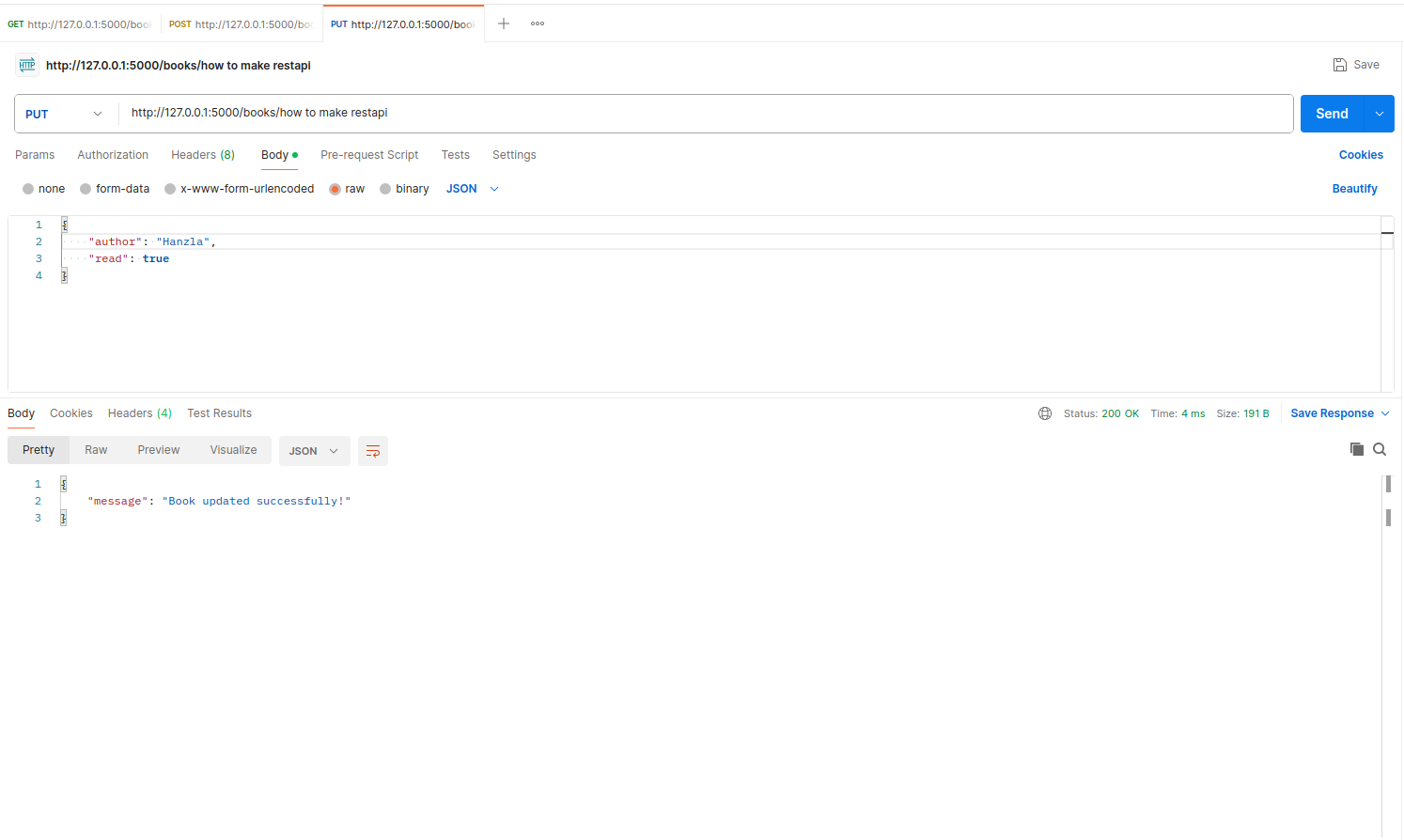
For ‘DELETE’ requests, request to delete the specified book by its ‘title’.
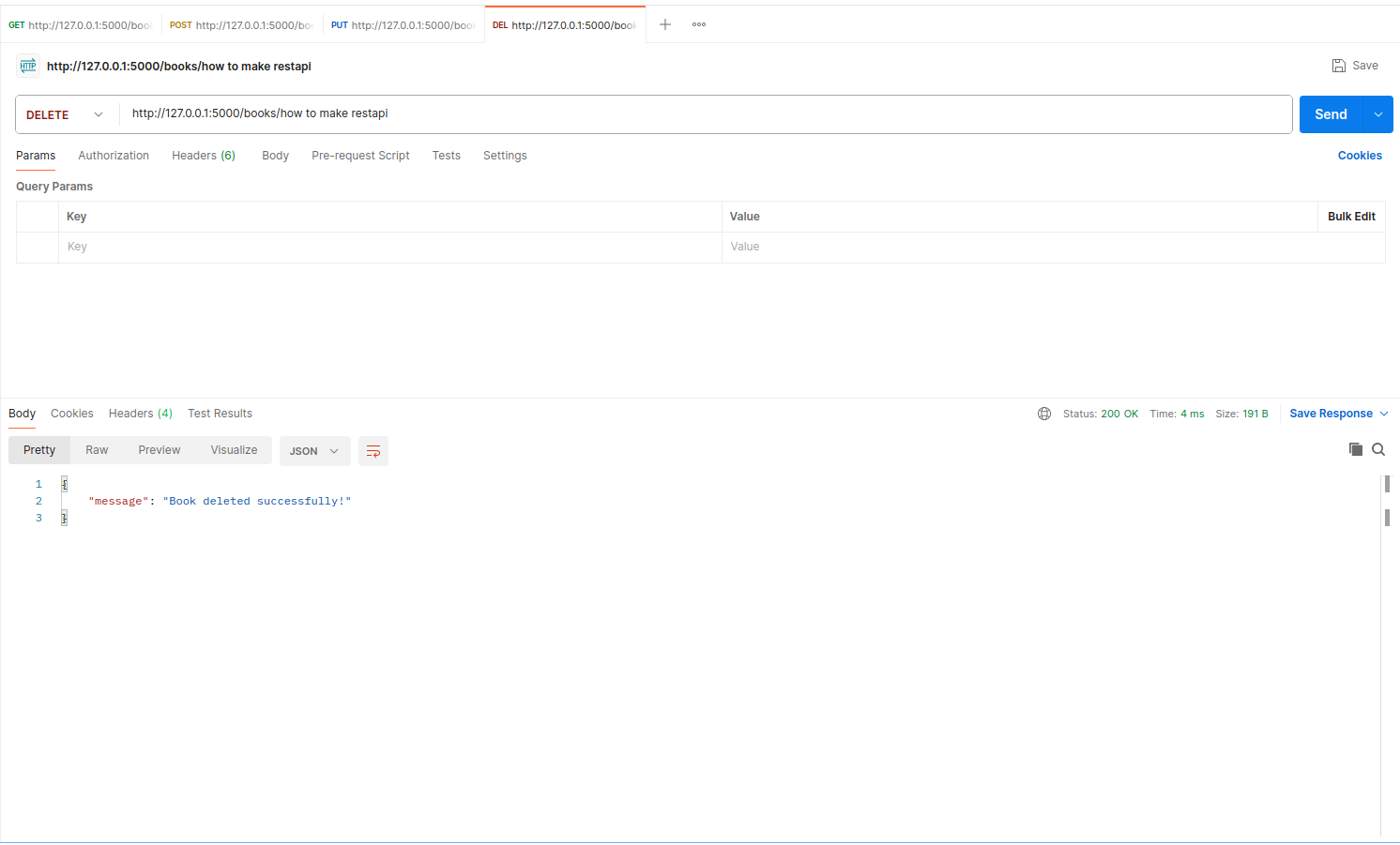
Understanding API Endpoints and Responses
GET /books: Retrieves all books. You’ll receive a JSON response with all the books.
POST /books: Adds a new book. You’ll receive a confirmation message in the response.
PUT /books/{title}: Updates an existing book. If the book exists, you’ll receive a success message; otherwise, a ‘Book not found!’ message.
DELETE /books/{title}: Deletes the specified book. If the book is found and successfully deleted, you’ll receive a success message; otherwise, a ‘Book not found!’ message.
And there you have it – a simple yet powerful REST API built with Flask.
Conclusion
Developing a REST API with Flask allows you to create scalable and versatile web services while leveraging the simplicity and power of Python. By following the steps outlined in this guide, you’ve learned how to set up a basic Flask project, handle HTTP methods, and create functional endpoints.
With your newfound knowledge, there’s no limit to what you can achieve with Flask and Python. Whether you’re building a small internal service for your team or a large-scale production API, sticking to RESTful principles will ensure your API is well-organized and easy to maintain. Keep practicing, and soon, you’ll master the art of building sophisticated REST APIs that cater to any application’s needs.
FAQS
SQLAlchemy is a popular database ORM (Object-Relational Mapper) for Flask apps, enabling easy integration with relational databases like MySQL and PostgreSQL.
Other popular web frameworks for Python include Django, Pyramid, and Bottle.
Yes, with the right extensions and design patterns, Flask can handle large projects effectively.
Flask, a popular Python framework, is used by major companies like Netflix, LinkedIn, and Airbnb. Its flexibility and scalability make it suitable for various projects, from small-scale personal projects to large-scale enterprise applications.
Flask is a versatile Python framework for building web applications and APIs. It’s lightweight, easy to learn, and suitable for a wide range of projects, from small prototypes to complex microservices.