Python has quickly become the go-to programming language for beginners and experienced developers alike. Its versatility, simplicity, and wide range of applications make it a powerful tool in the hands of programmers. Whether you’re just starting out or already familiar with Python or these Python tasks.
This blog will introduce you to 10 simple yet impactful tasks you can perform with Python for your personal or professional advancement. By the end of it, you’ll not only know how to leverage Python but also have actionable steps to elevate your coding skills.
Why is Python the Ultimate Tool for Coding?
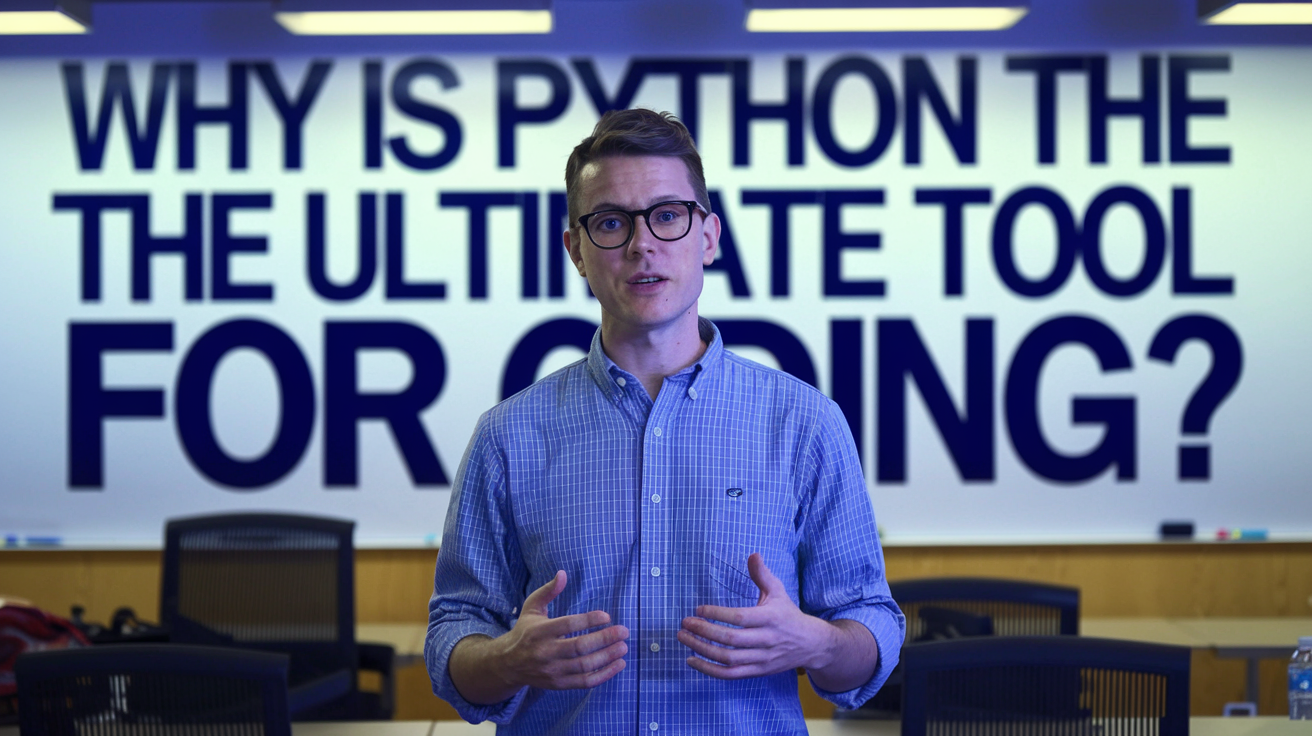
Python is a high-level, interpreted language designed for readability and ease of use. Its vast array of libraries and frameworks makes it ideal for tasks ranging from web development to machine learning, automation, and more. Plus, Python is open-source and supported by a massive, active community, so there’s no shortage of resources and guidance.
Now, let’s focus on 10 Python tasks that will accelerate your programming skills and show you just how versatile this language can be.
10 Simple Yet Powerful Python Tasks for Beginners and Advanced Users
Task 1: Data Manipulation with Pandas
Data manipulation is one of the most common tasks in programming—and Pandas makes it a breeze in Python. This library allows you to work with structured data (like tables or spreadsheets), conduct in-depth analyses, and clean data efficiently.
Example Use Case
Use Pandas for filtering a dataset to find trends or summarize customer data.
Key Libraries Needed
- pandas
Install libraries
pip install pandas
or
pip3 install pandas
Sample Code:
import pandas as pd
# Read a CSV file
data = pd.read_csv("sales_data.csv")
# Filter and summarize
filtered_data = data[data["Sales"] > 1000]
print(filtered_data.describe())
Task 2: Scraping Data from Websites Using Beautiful Soup
Data is gold, and with Python’s Beautiful Soup, you can extract data from websites effortlessly. Whether it’s gathering research data or monitoring competitors, web scraping is an excellent skill to master.
Example Use Case
Scrape headlines from a news website to analyze trending topics.
Key Libraries Needed
- BeautifulSoup4 (bs4)
- requests
Install libraries
pip install requests bs4
or
pip3 install requests bs4
Sample Code:
import requests
from bs4 import BeautifulSoup
# Send a request to the webpage
response = requests.get("https://newswebsite.com")
soup = BeautifulSoup(response.text, "html.parser")
# Extract headlines
headlines = soup.find_all("h2")
for headline in headlines:
print(headline.text)
Task 3: Machine Learning with Scikit-learn
Scikit-learn simplifies implementing machine learning models, even for beginners. From predictive analysis to building classification models, it’s the ideal library for learning and applying ML algorithms.
Example Use Case
Develop a machine learning model to predict house prices.
Key Libraries Needed
- scikit-learn
Install libraries
pip install scikit-learn
or
pip3 install scikit-learn
Sample Code:
from sklearn.datasets import load_boston
from sklearn.linear_model import LinearRegression
# Load data
boston = load_boston()
model = LinearRegression().fit(boston.data,boston.target)
# Check model Accuracy
print(model.score(boston.data,boston,target))
Task 4: Natural Language Processing with NLTK
The world of Natural Language Processing (NLP) opens doors to analyzing and understanding human language. With NLTK, you can perform sentiment analysis, tokenization, and more.
Example Use Case
Analyze customer reviews to identify their sentiment.
Key Libraries Needed
- nltk
Install libraries
pip install nltk
or
pip3 install nltk
Sample Code:
import nltk
from nltk.sentiment import SentimentIntensityAnalyzer
nltk.download("vader_lexicon")
sia = SentimentInetnsityAnalyzer()
# Analyze Sentiment
print(sia.polarity_scores("This product is amazing!"))
Task 5: Web Development with Flask or Django
Want to create a website? Python’s Flask and Django frameworks allow you to launch web applications with ease.
Example Use Case
Develop a personal website.
Key Libraries Needed
- Flask
Install Libraries
pip install flask
or
pip3 install flask
Sample Code with Flask:
from flask import Flask
app = Flask(name)
@app.route("/")
def home():
   return "Welcome to my website!"
if name == "main":
   app.run(debug=True)
Task 6: Data Visualization with Matplotlib and Seaborn
Visualizing data makes it easier to comprehend trends and insights. Python libraries like Matplotlib and Seaborn are perfect for creating stunning charts and graphs.
Example Use Case
Create a bar chart to compare product sales across regions.
Key Libraries Needed
- matplotlib
Install libraries
pip install matplotlib
or
pip install matplotlib
Sample Code:
import matplotlib.pyplot as plt
regions = ["North", "South", "East", "West"]
sales = [300, 400, 340, 450]
plt.bar(regions, sales)
plt.title("Product Sales per Region")
plt.show()
Task 7: Automation with Python Scripts
Python is ideal for automating everyday tasks like renaming files or processing text documents in bulk. Automation saves time, reduces errors, and improves efficiency.
Example Use Case
Rename multiple files in a folder with specific patterns.
Key Libraries Needed
- os
Sample Code:
import os
folder = "Documents"
for count, filename in enumerate(os.listdir(folder)):
   os.rename(filename, f"document_{count}.txt")
Task 8: Automating Email and SMS Notifications
With Python, you can automate communication, like sending emails or SMS alerts. Use SMTP for emails and services like Twilio for text messages.
Example Use Case
Send notifications to a list of users about upcoming sales.
Key Libraries Needed
- smtplib
- Twilio
Task 9: Working with APIs to Fetch and Update Data
APIs are essential for interacting with online services. Python makes working with APIs effortless through libraries like Requests.
Example Use Case
Fetch live weather data from a weather API.
Key Libraries Needed
- requests
Task 10: Building APIs with Flask-RESTful
Interested in creating your own API? Python’s Flask-RESTful library makes building APIs straightforward and robust.
Example Use Case
Develop a RESTful API for your app.
Key Libraries Needed
- Flask-RESTful
Popular Python Coding Examples For Beginners
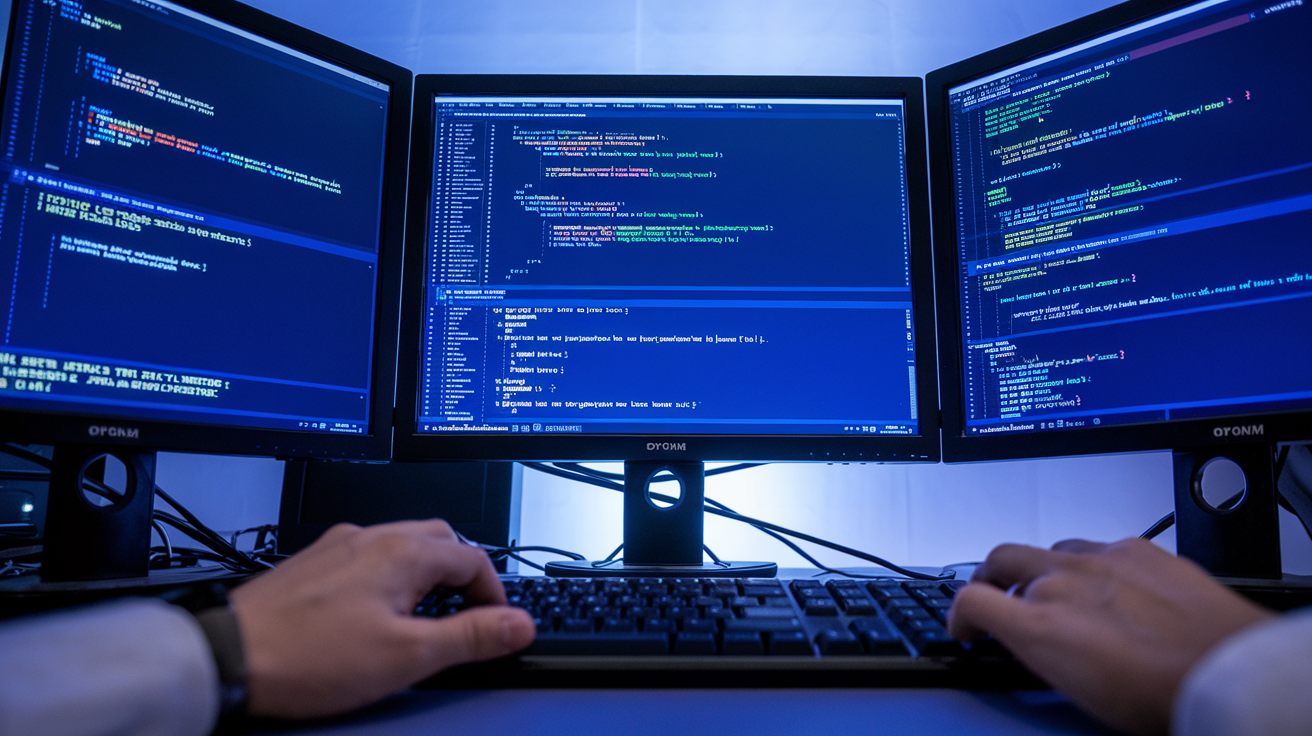
- Building a simple calculator
- Creating a to-do list app
- Writing a program to reverse text
- Developing a simple password generator
Best Resources and Tools for Further Learning
- Official Python Documentation
- Online Courses on platforms like Codecademy, Udemy, and Coursera
- Python Libraries such as NumPy, SciPy, and TensorFlow
Practice Regularly
Consistency is the backbone of mastering Python. Dedicate time daily or weekly to coding, exploring new libraries, and tackling real-world problems.
Contribute to Open Source
Give back to the programming community by contributing to open-source projects. Platforms like GitHub are a great place to start—plus, you’ll learn collaboration skills.
Final Thoughts: Your Next Steps in Python
Whether it’s automating your workflow, creating a stunning website, or building your first machine learning model, Python equips you to achieve your goals. And remember—practice is paramount. The more you code, the closer you’ll get to mastering Python.
Start small, stay consistent, and start advancing your coding knowledge with Python today!